Programs Using Loops
Q20.Write a program to display all the even numbers from 25 to 60.
Q21.Write a program to display all the odd numbers from 230 to 190 (in reverse order )
Q22.Write a program to display all the multiples of 37 from 380 to 600. (use while loop )
Q23.Write a program to accept a number then display its multiplication table. If the entered number is 5 then it should display as below-
5x1=5 5x2=10 . . 5x10=50Q24.Write a program to accept a number the display next 10 odd or even numbers.
Q25.Write a program to accept the range ( lower limit and upper limit) then display all the even or odd numbers within the range as per users choice
Program-20
#include <iostream.h> void main(){ int i; for(i=25; i<=60; i++){ if(i%2==0){ cout<< i <<" "; } } }In the above program note that we have generating all the numbers from 25 to 60 then we are checking if each number is divisible by 2, if yes we are displaying it as the number is even. In this proagram "if" statement is a time consuming statement if you can avoid it then your program will run faster, though is this small program it does not matter still you should do your best to make your program efficient one in all respect. Therefore let us write the same program without using the "if" statement. One more thing see that after the second pair of "<<" (output operator) a gap within quotes is used, it formats the output as 26 28 30 .... If it is not given the output will be as 262830... You can use "\n" which will display the output in seperate lines.
Program-20 (without if)
#include <iostream.h> void main(){ int i; for(i=26; i<=60; i=i+2){ cout<<i<<" "; } }In the above program see that the loop started with 26 not 25; since we have to display even numbers so starting even numbers within the range 25 to 60 is 26. Also see that the looping variable is incremented by 2 ( i=i+2 cab be written as i=+2) hence it will always generate the next even numbers. We should not worried about the upper limit wheather it even or odd, the looping statement will take care of it automatically
Program-21
#include <iostream.h> void main(){ int i; for(i=229; i>=190; i=i-2){ cout<< i <<" "; } }
Program-22
#include <iostream.h> void main(){ int i=407; while(i<=600){ cout<<i<<" "; i=i+37; } }In the above program see that the loop started with 407 which is the first multiple of 37 within the range 380 to 600, looping variable "i" is incremented by 37 to get the next multiple of 37.
Program-23
#include <iostream.h> void main(){ int i, a; cout<<"Enter the number="; cin>>a; for(i=1; i<=10; i++){ cout<< a << "x" << i << "=" << a*i << "\n"; } }Output of the above program as below
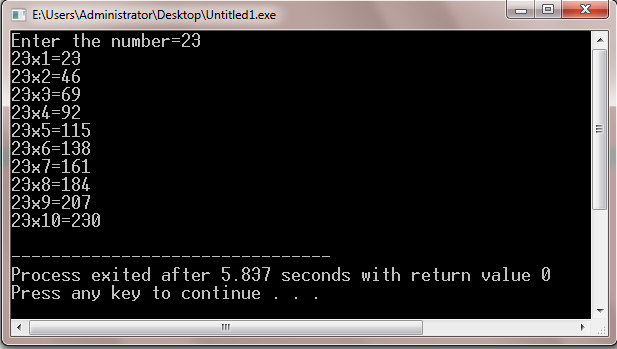
Program-24
#include <iostream.h> void main(){ int i, a; cout<<"Enter the number="; cin>>a; for(i=1; i<=10; i++){ cout<< a << " "; a=a+2; } }In the above see that the loop is used to count the number of numbers but not to generate the numbers. The numbers are being generated by the statement a=a+2
Program-25
#include <iostream.h> void main(){ int i, L, U, choice; cout<< "Enter the lower limit="; cin>>L; cout<< "Enter the upper limit="; cin>>U; cout<<"Enter 1 for odd numbers or 2 for even numbers="; cin>>choice; switch(choice){ case 1: if(L%2==0){ L=L+1; } for(i=L; i<=U; i=i+2){ cout<< i << " "; } break; case 1: if(L%2==1){ L=L+1; } for(i=L; i<=U; i=i+2){ cout<< i << " "; } break; default: cout<< "Sorry you have not choosen an allowed option !!!"; } }In the above program see that a variable "choice" is taken to accept the user choice ( even or odd ) by allowing him to choose 1 or 2 as per his need. If he chooses 1 then the "case 1" of the switch statement would execute and sililarly would execute "case 2", but if he chooses any other number he will get the message in the "default case". Now see that under "case 1" we have to generate odd number as per the option given, so we have to check if the lower limit (variable L ) is an even number, if yes then we have to start from the next number, so it is L=L+1. Same is the in case of even number we have to check if L is an odd number and treat in the same way. Take care of proper case (upper or lower) of the variable name because C++ is case sensitive
